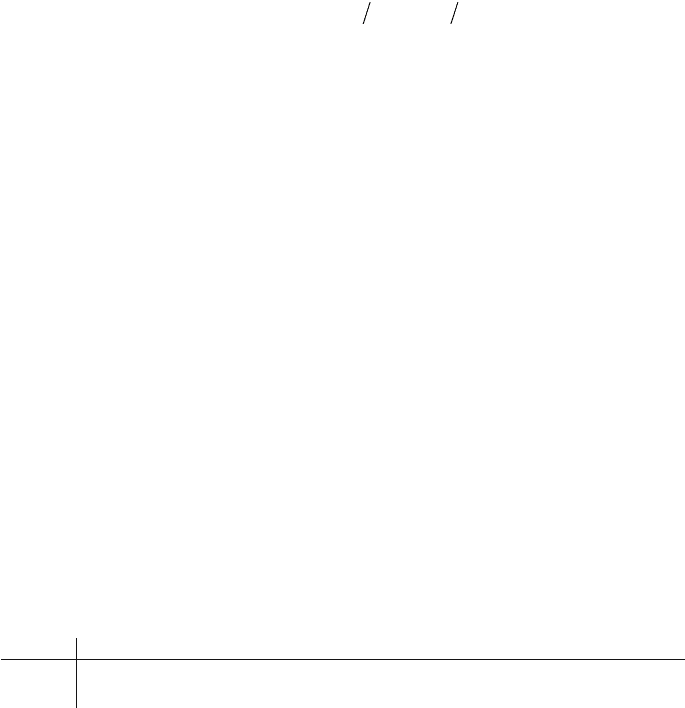
Suppose therefore that we want to draw a line from (0,0) to (a,b), where a and b
are integers and 0 £ b £ a (which puts (a,b) into the first octant). Using equation (2.3),
the points p
i
, 0 £ i £ a, generated by the simple DDA are then defined by
and the discrete line consists of the points (i,y
i
), where y
i
is the real number i(a/b)
rounded to the nearest integer.
Now the y coordinates start at 0. At what point does y
i
become 1? To answer this
question, we must compute b/a, 2b/a, 3b/a, ..., and watch for that place where these
values become bigger than 1/2. Furthermore, the y
i
will then stay 1 until these values
become bigger than 3/2, at which time y
i
will become 2. Since we want to avoid doing
real arithmetic, note that we do not really care what the actual values are but only
care about when they get bigger than 1/2, 3/2, 5/2, .... This means that we can mul-
tiply through by 2a and the answer to the question as to when y
i
increases by 1 is
determined by when 2b, 4b, 6b, . . . become bigger than a, 3a, 5a, .... Since comput-
ers can compare a number to 0 in less time than it takes to compare it to some other
number, we shall start off by subtracting a. Our first question now is
“When does 2b - a, 4b - a, 6b - a, . . . become bigger than 0?”
and only involves repeated integer additions of 2b to an initial sum d = 2b - a. After
the sum d has become bigger than 0 and y has switched to 1, we need to check when
the sum becomes bigger than 2a. By subtracting 2a, we again only need to keep check-
ing for when the sum gets to be bigger than 0 by successive additions of 2b. In general,
whenever y is incremented by 1, we subtract 2a from the current sum d. In that way
we always need to check d simply against 0. For example, suppose we want to draw
the line from (0,0) to (15,3). In this case, 2a = 30, 2b = 6, and the initial d is 6 - 15 =
-9. The table below shows the points (x
i
,y
i
) that are drawn and the changes to the
sum d as i ranges from 0 to 8:
i 012345678
d -9 -3 -27 -21 -15 -9 -3 -27
(x
i
,y
i
) (0,0) (1,0) (2,0) (3,1) (4,1) (5,1) (6,1) (7,1) (8,2)
The code in Algorithm 2.5.2.1 implements the algorithm we have been describing.
In our discussion above we have restricted ourselves to lines that start at the origin
and end in the first octant. Starting at another point simply amounts to adding a con-
stant offset to all the points. Lines that end in a different octant can be handled in a
similar way to the first octant case – basically by interchanging the x and y. What this
boils down to is that an algorithm which handles all lines is not much harder, involv-
ing only a case statement to separate between the case where the absolute value of
the slope is either larger or less than or equal to 1.
We have just described the basis for the Bresenham line-drawing algorithm
([Bres65]). It, or some variation of it, is the algorithm that is usually used for drawing
straight lines. Bresenham showed in [Bres77] that his algorithm generated the best-
fit discrete approximation to a continuous line. The variation that is Algorithm 2.5.2.2
pp
ii
ba iiba=+
()
=
()
-1
1, , ,
2.5 Generating Discrete Curves 39