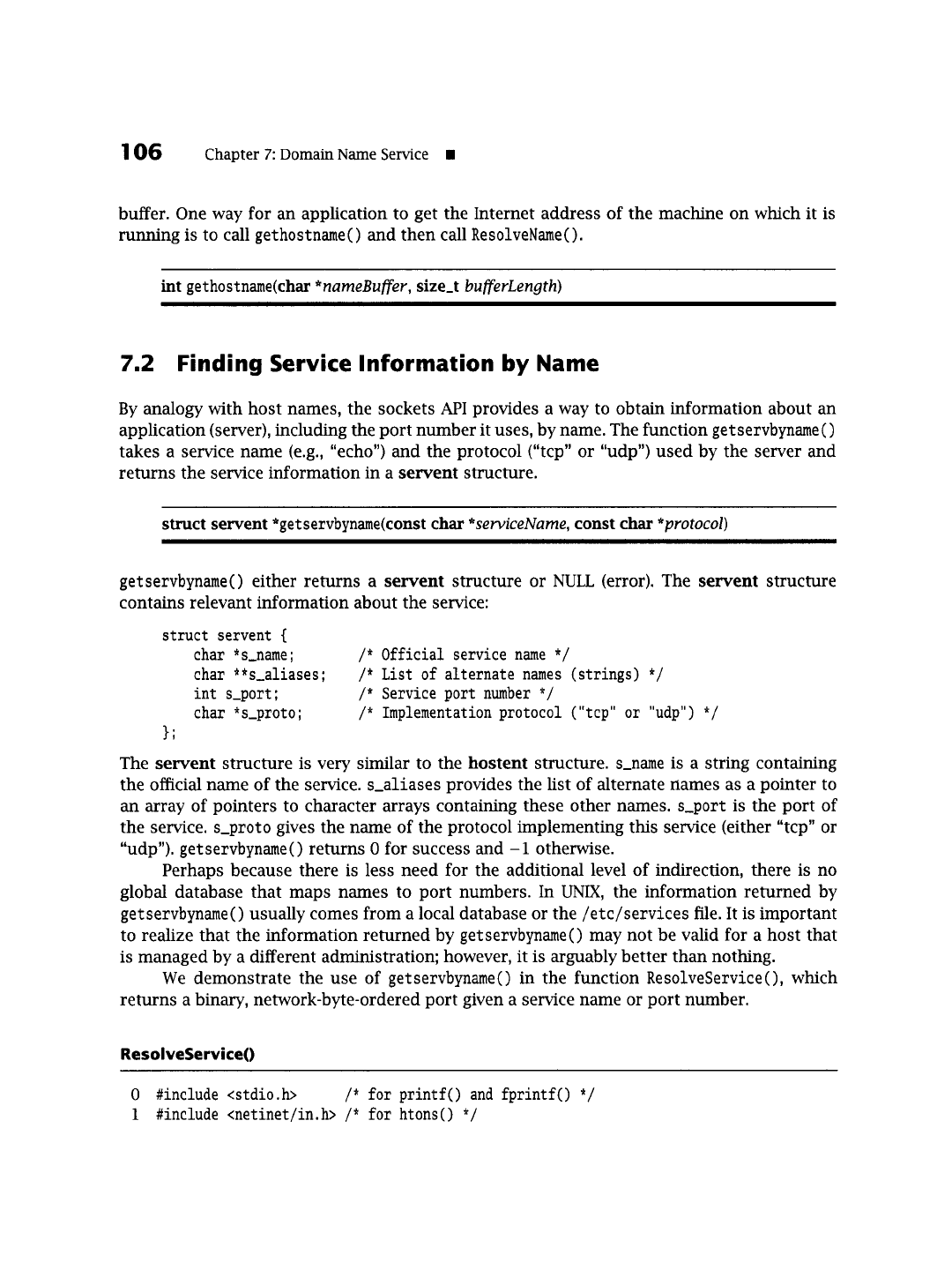
106
Chapter 7: Domain Name Service
m
buffer. One way for an application to get the Internet address of the machine on which it is
running is to call gethostname() and then call
ResolveName().
int gethostname(char *nameBuffer, size_t bufferLength)
7.2 Finding Service Information by Name
By analogy with host names, the sockets API provides a way to obtain information about an
application (server), including the port number it uses, by name. The function getservbyname()
takes a service name (e.g., "echo") and the protocol ("tcp" or "udp") used by the server and
returns the service information in a servent structure.
struct servent *getservbyname(const char
*serviceName, const char *protocol)
getservbyname() either returns a servent structure or NULL (error). The servent structure
contains relevant information about the service:
struct servent {
char *s_name;
char **s_aliases;
int s_port;
char *s_proto;
};
/* Official service name */
/* List of alternate names (strings) */
/* Service port number */
/* Implementation protocol ("top" or "udp") */
The servent structure is very similar to the hostent structure, s_name is a string containing
the official name of the service, s_aliases provides the list of alternate names as a pointer to
an array of pointers to character arrays containing these other names, s_port is the port of
the service, s_proto gives the name of the protocol implementing this service (either "tcp" or
"udp"). getservbyname() returns 0 for success and -1 otherwise.
Perhaps because there is less need for the additional level of indirection, there is no
global database that maps names to port numbers. In UNIX, the information returned by
getservbyname() usually comes from a local database or the/etc/serviees file. It is important
to realize that the information returned by getservbyname() may not be valid for a host that
is managed by a different administration; however, it is arguably better than nothing.
We demonstrate the use of getservbyname() in the function ResolveService(), which
returns a binary, network-byte-ordered port given a service name or port number.
ResolveServiceO
0 #include <stdio.h> /* for printf() and fprintf() */
1 #include <netinet/in.h> /* for htons() */