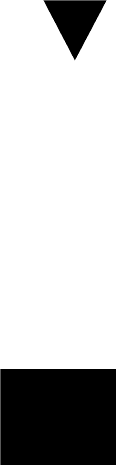
492
Chapter 21 Result Verification Patterns
This example includes plenty of conditional statements that the author might get
wrong—things like writing (foundPeople == null) instead of (foundPeople != null). In
C-based languages, one might mistakenly use = instead of ==, which would result
in the test always passing!
Refactoring Notes
We can use a Replace Nested Conditional with Guard Clauses [Fowler] refactoring
to transform this spider web of Conditional Test Logic into a nice linear sequence
of statements. (In a test, even a single conditional statement is considered too much
and hence “nested”!) We can use Stated Outcome Assertions to check for null
object references and Equality Assertions to verify the number of objects in the
collection. If each assertion is satisfi ed, the test proceeds. If they are not satisfi ed,
the test ends in failure before it reaches the next statement.
Example: Simple Guard Assertion
This simplifi ed version of the test replaces all conditional statements with asser-
tions. It is shorter than the original test and much easier to read.
public void testWithoutConditionals() throws Exception {
String expectedLastname = "smith";
List foundPeople = PeopleFinder.
findPeopleWithLastname(expectedLastname);
assertNotNull("found people list", foundPeople);
assertEquals( "number of people", 1, foundPeople.size() );
Person solePerson = (Person) foundPeople.get(0);
assertEquals( "last name",
expectedLastname,
solePerson.getName() );
}
We now have a single linear execution path through this Test Method (page 348);
it should improve our confi dence in the correctness of this test immensely!
Example: Shared Fixture Guard Assertion
Here’s an example of a test that depends on a Shared Fixture. If a previous test (or
even this test in a previous test run) modifi es the state of the fi xture, our SUT could
return unexpected results. It might take a fair bit of effort to determine that the
problem lies in the test’s pre-conditions rather than being a bug in the SUT. We can
avoid all of this trouble by making the assumptions of this test explicit through the
use of a Guard Assertion during the fi xture lookup phase of the test.
Guard
Assertion