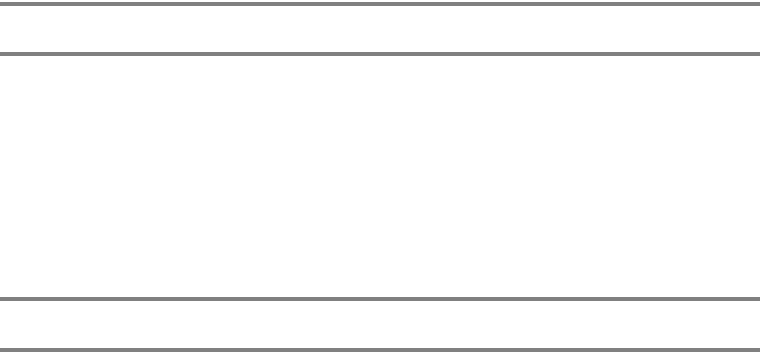
CHAPTER 3 ■ COLLECTIONS AND THE JOY OF IMMUTABILITY
67
a new Tuple3 by adding 1 to the first element of the Tuple, adding v to the second element
of the Tuple, and adding the square of
v to the third element of the Tuple. Using Scala’s
pattern matching, we can make the code a little more readable:
def sumSq(in: List[Double]) : (Int, Double, Double) =
in.foldLeft((0, 0d, 0d)){
case ((cnt, sum, sq), v) => (cnt + 1, sum + v, sq + v * v)}
You can create Tuples using a variety of syntax:
scala> Tuple2(1,2) == Pair(1,2)
scala> Pair(1,2) == (1,2)
scala> (1,2) == 1 -> 2
The last example, 1 -> 2, is a particularly helpful and syntactically pleasing way for
passing pairs around. Pairs appear in code very frequently, including name/value pairs
for creating
Maps.
Map[K, V]
A Map is a collection of key/value pairs. Any value can be retrieved based on its key. Keys
are unique in the
Map, but values need not be unique. In Java, Hashtable and HashMap are
common
Map classes. The default Scala Map class is immutable. This means that you can
pass an instance of
Map to another thread, and that thread can access the Map without
synchronizing. The performance of Scala’s immutable
Map is indistinguishable from the
performance of Java’s
HashMap.
We can create a
Map:
scala> var p = Map(1 -> "David", 9 -> "Elwood")
p: … Map[Int,String] = Map(1 -> David, 9 -> Elwood)
We create a new Map by passing a set of Pair[Int, String] to the Map object’s apply
method. Note that we created a
var p rather than a val p. This is because the Map is immu-
table, so when we alter the contents on the
Map, we have to assign the new Map back to p.
We can add an element to the
Map:
scala> p + 8 -> "Archer"
res4: … Map[Int,String] = Map(1 -> David, 9 -> Elwood, 8 -> Archer)
19897ch03.fm Page 67 Thursday, April 16, 2009 4:54 PM