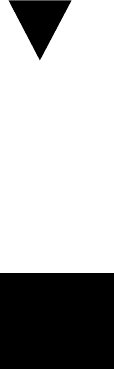
273
public void testRemoveFlight() throws Exception {
// set up
FlightDto expectedFlightDto = createARegisteredFlight();
FlightManagementFacade facade =
new FlightManagementFacadeImpl();
// exercise
facade.removeFlight(expectedFlightDto.getFlightNumber());
// verify
assertFalse("flight should not exist after being removed",
facade.flightExists( expectedFlightDto.
getFlightNumber()));
}
Note that this test does not verify that the correct logging action has been done.
It will pass regardless of whether the logging was implemented correctly—or
even at all. Here’s the code that this test is verifying, complete with the indirect
output of the SUT that has not been implemented correctly:
public void removeFlight(BigDecimal flightNumber)
throws FlightBookingException {
System.out.println(" removeFlight("+flightNumber+")");
dataAccess.removeFlight(flightNumber);
logMessage("CreateFlight", flightNumber); // Bug!
}
If we plan to depend on the information captured by logMessage when maintain-
ing the application in production, how can we ensure that it is correct? Clearly,
it is desirable to have automated tests verify this functionality.
Impact
Part of the required behavior of the SUT could be accidentally disabled without
causing any tests to fail. Buggy software could be delivered to the customer. The
fear of introducing bugs could discourage ruthless refactoring or deletion of
code suspected to be unneeded (i.e., dead code).
Root Cause
The most common cause of Untested Requirements is that the SUT includes
behavior that is not visible through its public interface. It may have expected
“side effects” that cannot be observed directly by the test (such as writing out
a fi le or record or calling a method on another object or component)—in other
words, it may have indirect outputs.
When the SUT is an entire application, the Untested Requirement may be a
result of not having a full suite of customer tests that verify all aspects of the
visible behavior of the SUT.
Production Bugs
Production
Bugs